What are loops for anyway?
With loops in JavaScript, we can repeat parts of code and control the flow of the programs. Most of the time this means that we repeat some kind of instructions for different cases.
Imagine that we need to bake 20 cakes we would not have a recipe that is made for 20 cakes but a single recipe, that we then repeat 20 times.
Loops are also great for counting, let’s say you need to show years from 1970 up to 2019, you can use a for loop for adding those elements.
Which loops in JavaScript do we have?
The list of loops in JavaScript is growing from year to year, most additions are made for easier use with array and other types of data. You should also take a look at our “What is JavaScript” article, just to cover all the basics.
Type of loops in JavaScript:
for
while
do/while
for/in
forEach
“loop” for arraysfor/of
All the types have their own purpose and we will explain them one by one, later on, we also have an example you can use on a simple web page.
for loop in JavaScript
for
loop is one of the fundamental loops in all the languages. It enables us to work with a numeric array, counting numbers,…
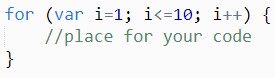
For loop has 3 parts: initialization, condition, and final-expression.
With the initialization part, we set the starting value for our loop variable; This is a variable that should change with every iteration. Many times i is used as a name for variable; but we encourage you to use more meaningful values like index, count,…. In fact, a name that makes your code more readable.
Condition is an important part of for loop since it makes sure that your loop will have an ending, condition when it stops iterating, looping…
Final-expression is a statement that will run at the end of the loop, after each iteration of the loop. Final-expression is in most cases some kind of increase/decrease of the loop variable. 99% of the time this code is equal to i++
which means that we increase i
by 1.
See example below to find out how to increase by two.
With for loop, we can:
Count from 1 to 100
- From 100 to 1
- Get all the even numbers between 1 and 100 (see example below)
- Get first 10 records from an array
- …
while loop in JavaScript
while
loop will loop through given code as long as a condition is true.
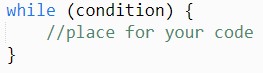
It has only “one” part, condition. A lot of times the value for the condition is changed within the loop itself.
While loop can be used for:
- Waiting for a condition to be true
- Increasing values till we reach some undefined value
- Searching for the end of the (data) tree
- …
You have to be careful with while loop, since a lot of times, developers make mistakes and the condition will never fire, this means that loop never ends. This kind of code then makes an application unresponsive, code is without error, but this is a runtime error and hard to find. To prevent this kind of error, you can add a counter, that breaks the loop after a number of loops.
do … while loop
do ... while
and while
loop are very similar, with one more important difference do … while will run at least once and it will run until the condition is true. (see the example with candies below to compare both types of loops)
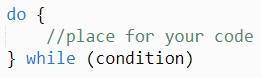
So what is this first run useful for? If we run a loop at least once, we can use the loop to initialize a condition, that we have to initialize before loop. Sometimes this makes the code cleaner and easier to control.
do…while vs. while loop
We all love candies, let me explain this with candies. 🙂
We have a condition that every Friday we give a piece of candy to all the employees, every hour. Other days we do not hand out candies, we all know that Fridays are special. This makes the do…while loop perfect for sweet tooth people.
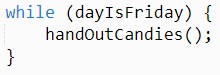
handOutCandies will only execute when the day is Friday and repeat every hour, we get 1 candy for a working week.
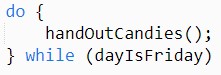
do…while will enable handOutCandies to execute every time we run the check, this means that we get at least one candy per day, or 5 candies a week.
I know you want more candies now, but this does not mean that you will always use the do…while loop, use it with care 😉
for…in loop in JavaScript
for...in
is an important loop for JavaScript since it loops through all enumerable properties of a JavaScript object. In other words, if we have an object we can read all the values the object has using the for...in
loop.

for...in
is very helpful if we have an object we know nothing about, and this enables us to shift through properties and make decisions. This happens a lot with server calls. Also, this makes using prototypes easier since we can make a loop not knowing all the properties.
for…in
can be mistaken for the for each loop which iterates through all the objects in an array. We still can use for...in
but it would return us indices of the given array, not the objects. The better loop would be the forEach
method of an array, or the for...of
loop.
forEach method of an array
forEach method is a “loop” that iterates all the objects in an array using a method. In order to use forEach we need to provide a function that consumes the object.
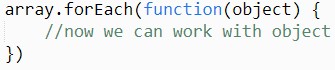
This is a very intuitive approach once you get used to it. You use an array as a base and a consume method, it returns all the needed objects. You don’t have to count, take care of edge cases or think about the types and values, just use the objects you expect.
Up till the newest JavaScript version, this is the preferred way, but the newest version introduced the for...of
loop. There is nothing wrong with forEach, but sometimes for…of makes usage more clean.
for…of loop
for...of
loop iterates through objects. For..of is a bit more powerful as it can iterate String
, Array
, array-like objects (e.g., arguments or NodeList), TypedArray, Map
, Set
, and user-defined iterables (a way to make a custom implementation of for..of loop).
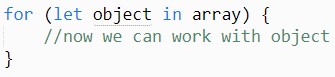
This is a code, that is similar to the one that we showed using the forEach method, each iteration gives us the possibility to work with object.
Example of how to use loops in JavaScript
It’s great to have more vivid example of how to use loops in JavaScript. We decided to write a simple web page that will showcase all the possible loops for you. If you want to create a more complex example take a look at our blog post How to create JavaScript list filter and Contact form in JavaScript.
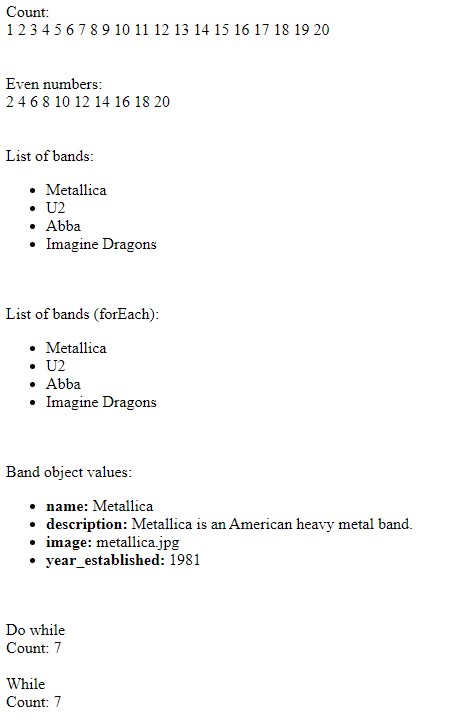
Our example has 2 parts; the web page (HTML) where all the visual components will be, and the JS file that will hold all the functions that will show the usage of loops.
We start with the web page, it’s a simple HTML document. Next, we add all the code inside the JavaScript file, the code will create all the content for the web page using loops.
Build a webpage to showcase all the loops in JavaScript
We need to start with a simple HTML template (we have one on our What is HTML blog post, check it out).
HTML to showcase loops
HTML
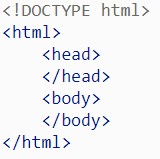
1. Add a Link to a CSS file
We need to connect the JavaScript file where we define all our functions and HTML.

If your file is named any different or is located in a subfolder you should change the value of the src
attribute.
2. Add elements for a simple count
From now on, we add all the visible elements inside the <body>
tag of the HTML document.
Div to show the counter
HTML
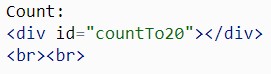
We add a label “Count:”, that is only a text inside the HTML, next we add a <div>
tag to store values. We add all the values inside the division (<div>) tag. We use id="countTo20"
for this tag so that we can find it in our code. The JavaScript code finds the element and changes the HTML for us with an array of numbers using the for loop.
After the div tags add two more <br>
tags to make some space
3. Add elements to show even numbers
We repeat similar steps for all examples of loops in JavaScript
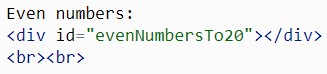
Be careful to use new values for id="evenNumbersTo20"
.
4. Add elements to list bands
We use a different element this time, an unordered list, or <ul>
tag. The label and brake stay the same.
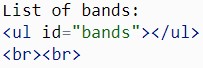
Using JavaScript we add <li>
tags to our unordered list, for every band we add a new list item.
We add a secondary list that we use to show the forEach method.
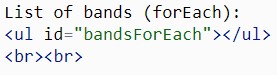
Don’t forget to change the id and label, so that you will know which list is which.
5. Add a list for band properties
Similar to the list of bands, we make a list of properties using an unordered list.
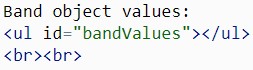
Add brakes here as well.
6. do…while (and while) counter
These elements will feature a simple counter, that will change every second
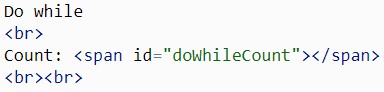
Since we showcase two things we have two labels one for the type of loop “Do while” or “While”

For counters we use the <span>
tag.
We have created an HTML document, here is the final code.
Final HTML code for loop examples
HTML
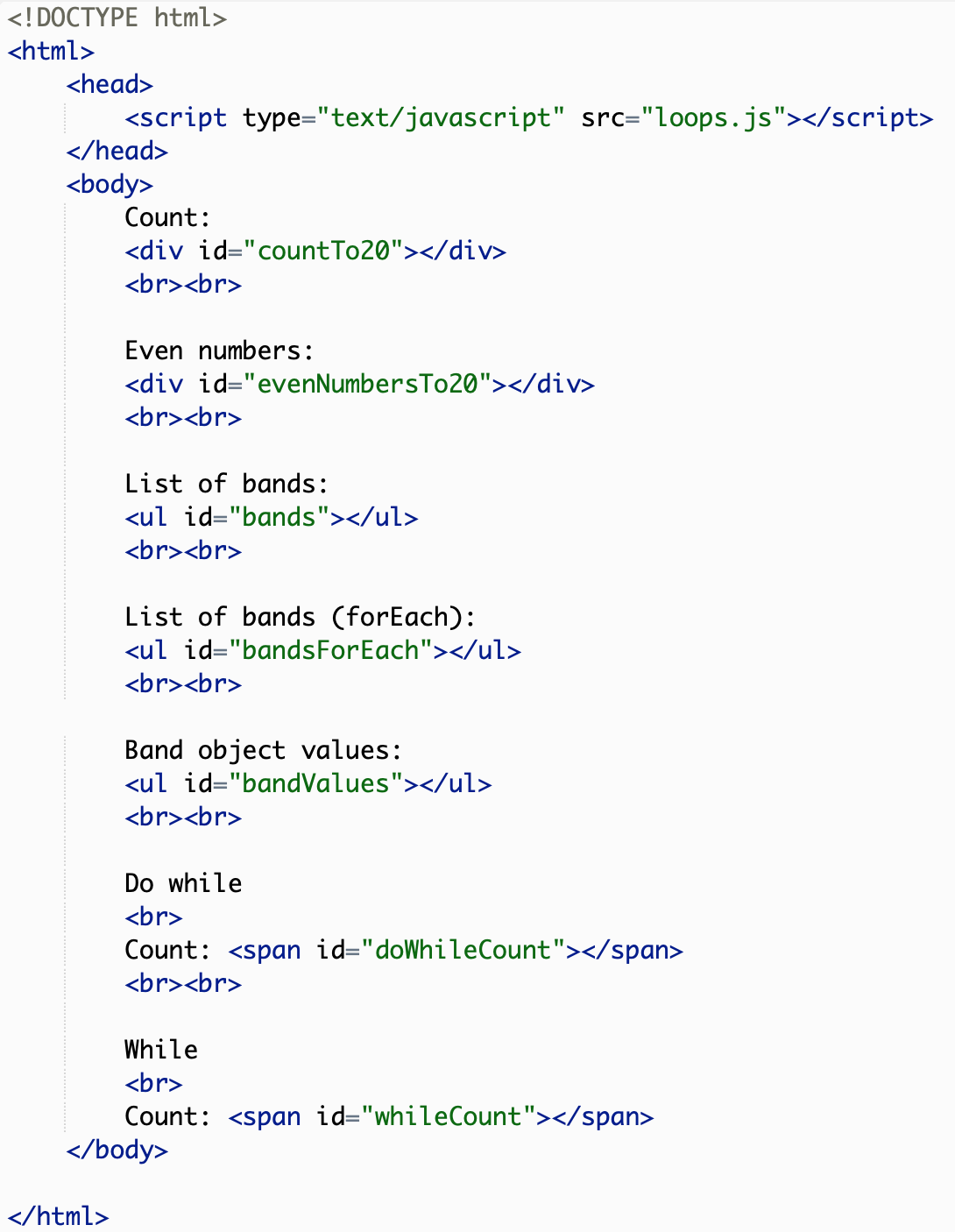
Let’s write JavaScript and show how to use loops
We have prepared the HTML document that will display all the values for us. It presents results for different loops we use in JavaScript.
It’s time to write some code and see the results. In this example we use simple data, but using loops with data from complex JSON source is practically the same. Check our blog post to see what is JSON data.
1. Counting to 20 using for loop in JavaScript
The first function we write is countTo20 as the name says it counts to 20, starting from 1.
Most of the functions we write have one variable at the beginning, this variable is meant to hold a reference to our element within DOM, this is the element, that shows the value on the screen. In our case we use getElementById
with id for countTo20
. Read more about DOM in JavaScript.
Using for loop to count to 20
JavaScript
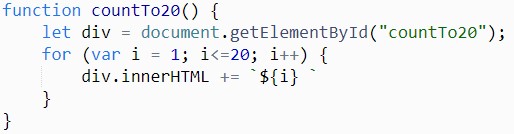
The following variable is our for loop. Now we can see all the parts in action. We initialize variable i
with 1. Next, we want to count till 20, this means that the variable i needs to be smaller or equal to 20. In the end we need to increase the value of i with every iteration.
We set the value to innerHTML, this is the property that changes the text that is displayed.
For the value we use string literals, this means that we use a backtick ` instead of a normal apostrophe. We use backticks to enable usage of variables inside the text ${}
.
2. Even numbers, changing the step with for loop
With this function, we show how we can change the way the loop increases the value. This is a good way to display even numbers.
If you compare it to the counting function, all that changes is that the variable i++ becomes i+=2, which means that we take the value of the variable i and add 2.
Use for loop and step by 2 to show even numbers
JavaScript
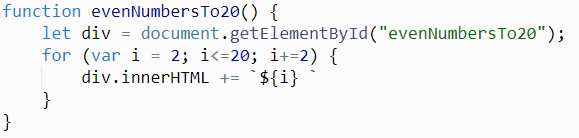
We use backticks here as well.
3. Using for…of loop and forEach method in JavaScript
Here is how to make a list of bands from an array, that is stored in a variable named bands
.
We need a variable named ul for our unordered list. Furthermore, we also need to create an array, this will be the list of bands. We can initialize the list with square brackets ([]) and then just add names you want, just make sure to separate them with commas.
Using the for..of
loop we can now work with each band name. Inside the loop we create a new list item using document.createElement("li")
. Next, we set the value using innerHTML
, at the end we need to update the list using appendChild
.
for of loop example
JavaScript
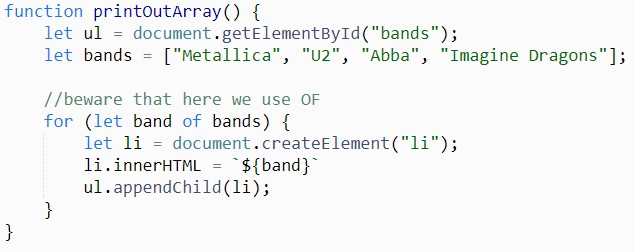
The code below is a comparison of how you can implement the same functionality as before but using the array.forEach
method.
The forEach method requires a function that has one parameter for our object from the array, we name it band as in the for..of loop.
forEach loop example
JavaScript
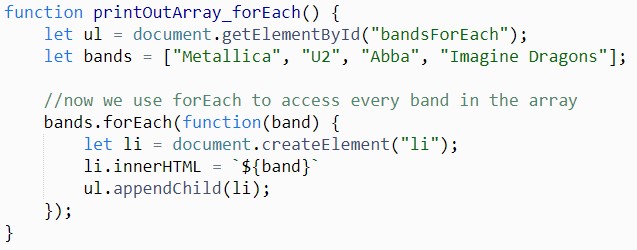
4. Get object properties with for…in loop
Working with objects is a big part of programming, and sometimes we do not know anything about an object we have. For example, when we receive an object from a server or any other source. In that case, it is very useful to have a way to go through properties of that object.
In our case we create our object, that will check properties. This is our sampleObject
, it has a description of a band. We added a minimal set of properties that describes a band, but you can go wild and add some more.
for .. in loop example
JavaScript
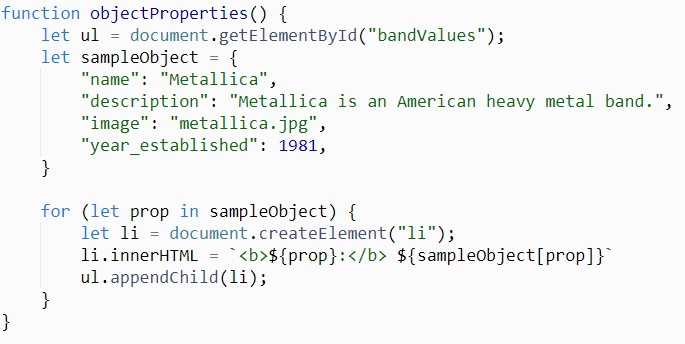
When we have an object, we can use the for…in loop and print out properties of that object.
Within the loop, we create a new list item and add it to our list. We go a bit crazy here and add some style, we make the name of the property bold, so it will look nicer on our web page.
5. do…while and while loops – with a counter example
Even though do…while and while loops are more standard than not we kept them to the end. We want to show you a simple counter. For that, we need a few special things.
We need to mark our functions with async, that means that functions will run in a separate thread and will not stop the main thread from executing. This means that we do not need to wait for them to execute something.
We want the async functions so that we execute them when the page loads, but they run for a longer time. For 10 seconds to be exact.
Next we need a simple way to simulate a 1 second delay. For that, we need a function called sleep. It creates a newTimeout and we then wait for it to execute. At this point, you can just go with the flow and use it. We promise that it will look cool on screen.
Delay simulation for examples
JavaScript

At last, we use loops.
First, we use a while loop. We get our span with the id whileCount and change its value for every iteration. At the end of the iteration, we call the sleep
function from above.
While loop example
JavaScript
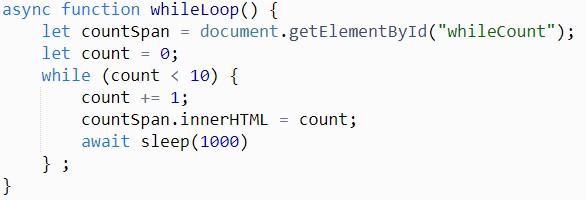
The do while loop should do the same. But we use different id-s to have two counters on screen.
Do while loop example
JavaScript
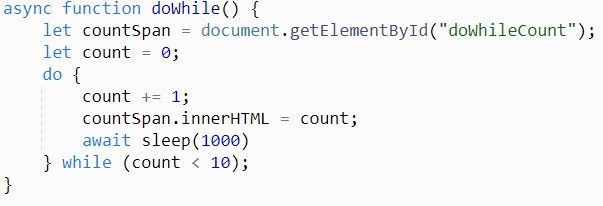
Remember the do while loop always runs once, so it is good for initialization. For practice you can change the conditions and use (count > 0 and count < 10)
. You will see the difference there.
6. Running all the code
We added all the code, now we need to combine all functions and execute them when the document loads. We add a new function called runAll. All this function does, that it calls all other functions we have.
Make all the calls for loops
JavaScript
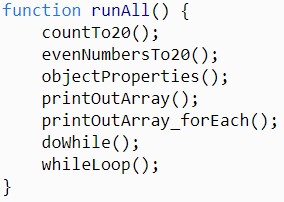
If we want to execute a piece of code when the HTML document loads we need a bit of fancy code.
document.addEventListener is a way to tell our web page that we want to listen for an event, and that event is when the page finishes loading. This event is called DOMContentLoaded. If this event loads we run our runAll function (be careful, no brackets after runAll, we are just linking a function and event, not executing the function)
Use loops in JS eventListener
JavaScript

Conclusion on how to use loops in JavaScript
We wanted to show you how to use loops in JavaScript in different ways. Loops are part of programming in a big way, even though you can only use the standard for
loop, the way you iterate keeps changing, to make our lives easier.
If you have any questions or remarks please post them below. So that we at CodeBrainer can improve our blog for you even further.